728x90
반응형
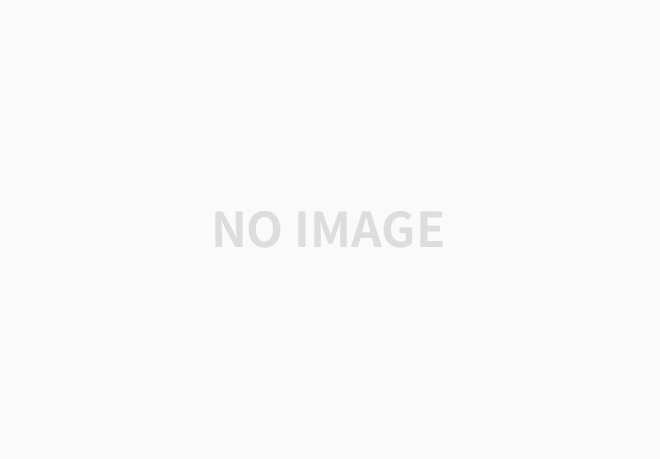
이 글은 아래 영상을 참고했다.
https://www.youtube.com/watch?v=CYmVcjIBlsM&list=PLz2iXe7EqJOMp5LozvYa0qca9E4OBkevW&index=5
이번 시간에는 이미지를 불러오고, 화면에 적용시키는 방법을 배웠다.
import pygame
이미지 로드하기 및 배치하기
# 이미지 불러오기(load)
pygame.image.load('이미지 파일 경로')
# 이미지의 사이즈 가져오기(배경)
background.get_size()[0] # 가로
background.get_size()[1] # 세로
# 이미지의 사이즈 가져오기(객체)
이미지.get_rect().size[0] # 가로
이미지.get_rect().size[1] # 세로
# 이미지 적용하기
background.blit(이미지, 좌표)
pygame.init()
background = pygame.display.set_mode((818, 532)) # 배경 이미지에 맞추어 화면크기 설정함
pygame.display.set_caption('PYGAME_4')
# 이미지 파일 준비
image_bg = pygame.image.load('./image/stadium.png')
image_ball = pygame.image.load('./image/ball_small.png')
image_player = pygame.image.load('./image/player_small.png')
# 배경의 사이즈 가져오기
size_bg_width = background.get_size()[0]
size_bg_height = background.get_size()[1]
# ball의 사이즈 가져오기 (가로, 세로)
size_ball_width = image_ball.get_rect().size[0]
size_ball_height = image_ball.get_rect().size[1]
# ball의 좌표값 설정하기
x_pos_ball = 10 # x좌표 상 왼쪽 살짝 앞에 위치
y_pos_ball = size_bg_height/2 - size_ball_height/2 # y좌표 상 가운데 위치
# player의 사이즈 가져오기
size_player_width = image_player.get_rect().size[0]
size_player_height = image_player.get_rect().size[1]
# player의 좌표값 설정하기
x_pos_player = size_bg_width - size_player_width # x좌표 상 제일 끝(오른쪽)에 위치 / 가려지지 않도록 조정
y_pos_player = size_bg_height/2 - size_player_height/2 # y좌표 상 가운데 위치
play = True
while play:
for event in pygame.event.get():
if event.type == pygame.QUIT:
play = False
# 이미지 삽입시에는 background.blit(이미지, 좌표) 사용
background.blit(image_bg, (0,0))
background.blit(image_ball, (x_pos_ball, y_pos_ball))
background.blit(image_player, (x_pos_player, y_pos_player))
pygame.display.update() # 업데이트 잊지 말기!
pygame.quit()
코드 실행 결과
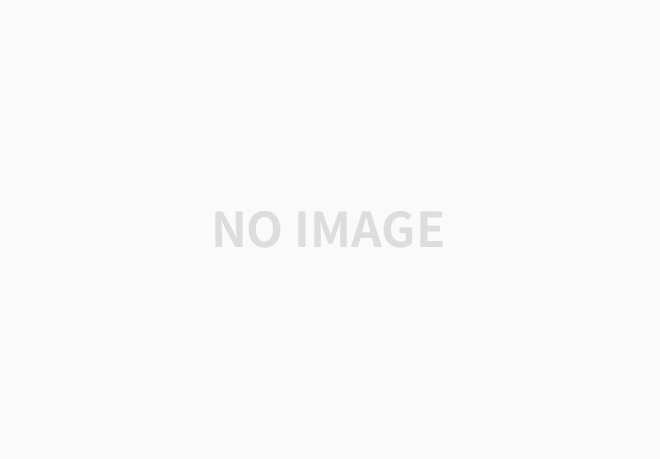
- 이미지의 중심좌표가 아닌 왼쪽 가장자리(0,0)를 기준으로 배치하게 됨
- ex) 이미지를 x좌표 상 가운데 두고 싶다면
- 배경의 width/2에서 이미지의 width/2만큼을 빼주어야 이미지를 가운데 위치시킬 수 있다.
728x90
'Minding's Programming > Pygame' 카테고리의 다른 글
[Pygame] 07. 이미지 벽에 닿았을 때 튕기기 (0) | 2023.02.05 |
---|---|
[Pygame] 06. 키보드와 마우스로 이미지 움직이기 (0) | 2023.02.04 |
[Pygame] 04. 도형 그리기 (0) | 2023.01.31 |
[Pygame] 03. 마우스로 조종하기 (0) | 2023.01.30 |
[Pygame] 02. 키보드로 조종하기 (0) | 2023.01.27 |